Custom Artisan command: Create one
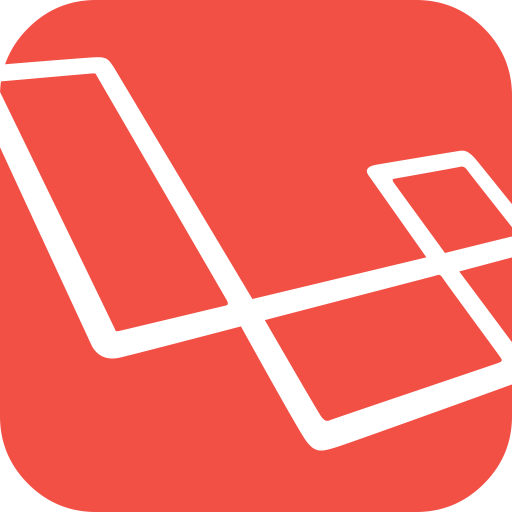
Artisan is the command-line interface included with Laravel, driven by Symfony Console component. It provides an awesome set of useful and most often used commands to help in app development with Laravel. But it includes the common and widely used command set only, that sometime might not be enough for our app development. For such conditions, we can create custom artisan command ourselves, as per our requirement.
Create custom command
Artisan provides an artisan command make:command to create the custom artisan command. This command create a basic boilerplate for creating custom artisan command. To initiate a create custom command, we run following command:
$ php artisan make:command MakeCustomCommand --command=make:custom
Here, php artisan make:command is predefined artisan command that facilitates in creating new artisan command. –command=make:custom is the command we want to run, that tells the app to perform certain operation we defined. And MakeCustomCommand is the file name that is created inside ProjectRoot/app/Console/Commands/ folder, where we define the operations/actions to be performed when we run our custom command. The above command will create a file MakeCustomCommand.php with following content.
<?php
namespace App\Console\Commands;
use Illuminate\Console\Command;
class MakeCustomCommand extends Command
{
/**
* The name and signature of the console command.
*
* @var string
*/
protected $signature = 'make:custom {name}';
/** * The console command description. * * @var string */ protected $description = 'Command description'; /** * Create a new command instance. * * @return void */ public function __construct() { parent::__construct(); } /** * Execute the console command. * * @return mixed */ public function handle() { // }
}
All the actions to be performed are defined inside the handle() method.
Print Output
To return output to the console, we can use any of these methods : line, info, comment, question and error methods. These methods display output in an appropriate ANSI colors depending on their purpose.
$this->line("Some text"); $this->info("Hey, watch this !"); $this->comment("Just a comment passing by"); $this->question("Why did you do that?"); $this->error("Ops, that should not happen.");
Arguments & Options
We can also pass arguments with the custom artisan command and access those in our command. The arguments are registered in our command with{argumentName}
and the options as {--optionName=}
. Thus, we don’t have to create the get options and get arguments methods in our class.
- Argument:
make:custom {argument}
- Optional Argument:
make:custom {argument?}
- Argument with default value:
make:custom {argument=defaultValue}
- Boolean Option: make:custom
--option
- Option with Value: make:custom
--option=
- Option with Value and Default: make:custom
--option=12
$ php artisan make:custom {argument1} {argument2?} {--option1=} {--option2=defaultValur}
Accessing arguments and options inside command
public function handle() { // Get All Parameters $arguments = $this->argument(); $options = $this->option(); // Get Single Parameters $username = $this->argument('username'); $enable = $this->option('enable'); }
Execute from Controller or Route
$commandExecute = Artisan::call('make:custom', [ 'username' => 'Carlos', '--enable' => true ]);
For more detailed documentation, check here