Best practice for writing readable code
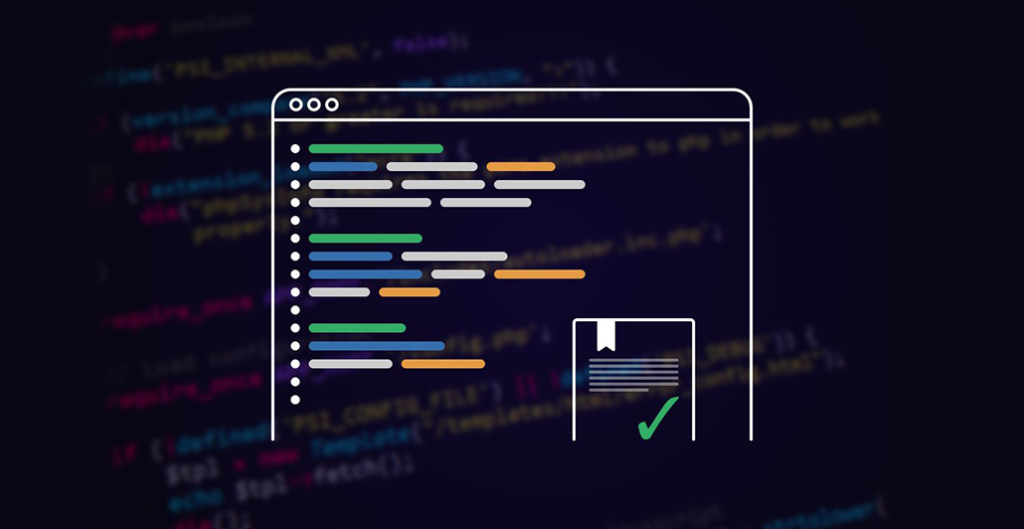
Clean and maintainable code is one of the fundamentals of development. Code readability also helps in team development. Each team members can understand and maintain the code written by fellow team members. Best practice for writing readable code doesn’t mean everyone should follow it as it is. Different teams may have their own pattern of coding practice. Here are few best practices to write readable code.
Consistent Naming Scheme
There are two major naming scheme practices:
- camelCase: The first letter of each word of variable name is capitalized, except the first word. eg: myVar or myFunction()
- underscores_name: Underscores between words. eg. my_var or my_function()
There is no hard n’ fast rule to follow certain pattern. Developers can choose or create their own patter as naming scheme but the best practice would be to follow certian conventions that project already has been folllowing. We can also choose to mix different patterns depending on the context of use; such as: camelcase for function names and underscores for variable names.
class My_Class {
public my_variable;
function myFunction() {
// do something
}
}
Avoid Deep Nesting
Avoid multiple levels of nesting as it makes harder to read and understand.
...
if (is_user_active(userId)) {
if (has_access_to_edit(userId)) {
if (is_owner_of_post(postId)) {
if (do_some_stuff()) {
// do domething
} else {
return false
}
} else {
return false
}
} else {
return false
}
} else {
return false
}
...
Some changes to above code can optimize readability and logical clearity by reducing nesting levels.
...
if (!is_user_active(userId)) {
return false
}
if (!has_access_to_edit(userId)) {
return false
}
if (!is_owner_of_post(postId)) {
return false
}
if(stuff = do_some_stuff()){
// do something
}
else{
return false
}
...
Comments and Documentation
Code comments are a kind so self documented code that explains the functionality and dependencies in plain language. Those comments avoid the requirement to refer to developer who created that code, for any case of update or maintenance. Any fellow developer can understand and take on further development based on it.
...
/**
* Get population for given country
/*
function country_population(country){
// do something
return Math.floor(1 + Math.random()*(99999999 - min + 1))
}
...
Comments added to the function definition can also be utilized by IDEs and code editors for code hinting.
But do avoid obivious commets as it’s really not productive and redundant when code is obivious and self explaining.
// get a random number
number = get_random_number()
// check if number is equal to 100
if(number === 100){
// display a message
print "Message"
}
On another thought, it can be simplified to describe the process
// show message if number is equal to 100
number = get_random_number()
if(number === 100){
print "Message"
}
Code comments should explain what and why we are doing something the way we did it.
Indentation
Indenting code adds clear visual to code block organization.