React Redux application npm
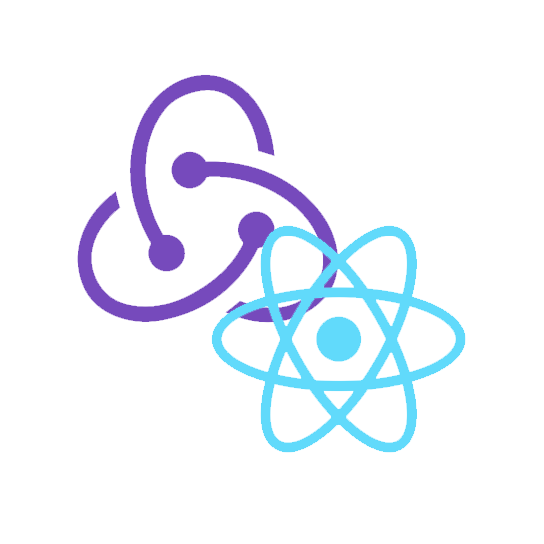
React is a javascript library for build User Interfaces. Redux is an opensource javascript library for managing application state. React app has a lot of states to maintain. It is feasible for small apps. But when it gets bigger and add up more behaviours, it gets tricky and dirty. For such cases, Redux comes as solution. Redux helps to give exact state data required by the app components. Here we are going to build a simple react redux app.
Create new ReactJs app
create-react-app react-redux-app cd react-redux-app npm start
We just created a very basic react app running with the basic template given by create-react-app.
Here we are creating simple app to toggle the content on click. So, let’s first add required redux package to our project.
Including React Redux Package
npm install --save redux react-redux
To manage our app and keep our app sttructure clean, we will organize our code in respective directory structure.
| react-redux-app | - src | - - actions | - - reducers
Actions
As we are going to change the content on click action, we are going to need a click action.
mkdir src/actions touch src/actions/clickAction.js touch src/actions/index.js
Now in clickAction.js
export const clickAction = { type: "click", payload: true };
In src/sctions/index.js,
export * from './clickAction';
Reducers
We will tell our reducer about the click type action and the state of the content should change. The reducer is a pure function that takes the previous state and an action, and returns the next state. Let’s create our reducer
mkdir src/reducers touch src/reducers/clickReducer.js touch src/reducers/index.js
Our reducer is just a bunch of switch case statements that returns the next state depending on the action. Our clickReducer.js will look like following.
export default (state, action) => { switch (action.type) { case "click": return { clicked: action.payload }; default: return state; } };
And in index.js file under reducers directory.
import { combineReducers } from 'redux' import click from "./clickReducer" const reducers = combineReducers({ click:click }) export default reducers
This is very useful when we have more that one reducer.
Redux Store
One thing we need to do now is create a store for our app. In src/index.js, import required packages and create store.
import {createStore} from 'redux' import {Provider} from 'react-redux' import reducers from './reducers' export const store = createStore( reducers );
And wrap our App component with react redux Provider component, instead of rendering App component directly
ReactDOM.render(<Provider store={store}><App/></Provider>, document.getElementById('root'));
App Components
Now inorder to access redux store from our app component, we first neet to connect our component to redux store. For that, we import connect from react-redux.
import {connect} from 'react-redux'
And export our App component through connect method.
export default connect()(App)
To retrive the state form our store, we will use connect method that accespts two parameters.
- mapStateToProps: To retrieve store state
- mapDispatchToProps: To retrieve actions and dispatch to the store
Let’s define the arguments required for connect method outside the App component class.
import {bindActionCreators} from 'redux' import {clickAction} from './actions' function mapStateToProps(state) { return { ...state }; } function matchDispatchToProps(dispatch) { return bindActionCreators({ clickAction }, dispatch) }
And Finally, we export our App component as follows:
export default connect(mapStateToProps,matchDispatchToProps)(App);
App Component
Our App Component, will look like follows.
import React, {Component} from 'react' import {connect} from 'react-redux' import {bindActionCreators} from 'redux' import './App.css'; import {clickAction} from './actions' class App extends Component { render() { let text = this.props.click.clicked ? "Clicked!" : "Click Me!" return ( <div className="App"> <h1 onClick={e => this.props.clickAction(!this.props.click.clicked)}>{text}</h1> </div> ); } } function mapStateToProps(state) { return { ...state }; } function matchDispatchToProps(dispatch) { return bindActionCreators({ clickAction }, dispatch) } export default connect(mapStateToProps, matchDispatchToProps)(App)
Now run app with npm start if not run yet, then go to browser. We will see our page with the following content. On clicking on the text, the content will toggle as “Clicked!” and “Click Me!”.
The complete code of app is available here.
We are just getting started with React and Redux. Keep exploring 🙂